The /account API calls
Upgrade to new version
You are viewing old calls for version 2011-01-15. Please upgrade account object calls to version 2014-01-08 for latest functionality.
The "account" object represents a payment account for accepting payments. The following calls let you create, view, and
modify "account" objects on WePay:
Account States
The "account" object has the following states and the following possible state transitions
(you can receive callback notifications when the account changes state, please read our
IPN Tutorial for more details):
- active
- The account is active and ready to accept payments.
- disabled
- The account has been disabled by WePay and can no longer accept payments.
- deleted
- The account has been deleted.
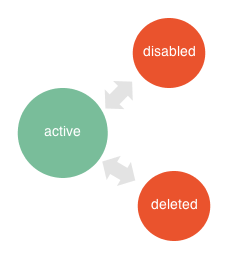
/account
This call allows you to lookup the details of a payment account on WePay.
The payment account must belong to the user associated with the access token used to make the call.
Arguments:
Parameter |
Required |
Type |
Description |
account_id |
Yes |
Integer (64 bits) |
The unique ID of the account you want to look up. |
Example:
{
"account_id":12345
}
Response:
Response |
Type |
Description |
account_id |
Integer (64 bits) |
The unique ID of the account. |
name |
String (255 chars) |
The name of the account. |
state |
String (255 chars) |
The state of the account: active, disabled or deleted. |
description |
String (65535 chars) |
The account description. |
reference_id |
String (255 chars) |
The unique reference ID of the account (this is set by the application in the /account/create or /account/modify call). |
account_uri |
String (2083 chars) |
A uri that corresponds to the account's page on WePay. Do not store the returned URI on your side as it can change. |
payment_limit |
Decimal (64 bits) |
The maximum recommended amount in dollars (including fees) for any payment to this account. A payment above this limit will go through but will get extra scrutiny from WePay's Risk Engine. |
gaq_domains |
Array |
An array of Google Analytics domains associated with the account. See the analytics tutorial for more details. |
theme_object |
Object |
The theme object you want to be used for account's checkout flows, withdrawal flows, and emails. See the customization tutorial for more details. |
verification_state |
String (255 chars) |
"unverified" if the account has not been verified and the verification data has not been collected. "pending" verification state means that the data was collected and no further action is required by the app. "verified" state is returned if the account is verified. |
verification_uri |
String (2083 chars) |
If the account is "unverified" then you can send the user to this url to verify their account. Do not store the returned URI on your side as it can change. |
type |
String (255 chars) |
The account type. Can be "personal", "nonprofit", or "business". |
create_time |
Integer (64 bits) |
The unixtime when the account was created. |
country |
String (2 chars) |
The account's country of origin 2-letter ISO code (e.g. 'US') |
currencies |
Array |
Array of supported currency strings for this account (e.g. ["USD"]). Only "USD" is supported for now. |
Example:
{
"account_id":12345,
"name":"Example account",
"state":"active",
"description":"this account is just an example.",
"reference_id":"123abc",
"account_uri":"https://stage.wepay.com/account/12345",
"payment_limit":2000,
"verification_state":"pending",
"type":"personal",
"create_time":1367958263,
"country": "US",
"currencies": ["USD"]
}
/account/find
This call lets you search the accounts of the user associated with the access token used to make the call.
You can search by name or reference_id, and the response will be an array of all the matching accounts.
If both name and reference_id are blank, this will return an array of all of the user's accounts.
Arguments:
Parameter |
Required |
Type |
Description |
name |
No |
String (255 chars) |
The name of the account you are searching for. |
reference_id |
No |
String (255 chars) |
The reference ID of the account you are searching for (set by the app in in /account/create or /account/modify). |
sort_order |
No |
String (255 chars) |
Sort the results of the search by time created. Use 'DESC' for most recent to least recent. Use 'ASC' for least recent to most recent. Defaults to 'DESC'. |
Example:
{
"name":"Example Acccount",
"reference_id":"123abc"
}
Response:
An array of accounts matching the search parameters. Each element of the array will include the same data as returned from the /account call.
Example:
[
{
"account_id":12345,
"name":"Example account",
"state":"active",
"description":"this account is just an example.",
"reference_id":"123abc",
"account_uri":"https://stage.wepay.com/account/12345",
"payment_limit":"2000",
"verification_state":"pending",
"type":"personal",
"create_time":1367958263,
"country": "US",
"currencies": ["USD"]
}
]
/account/create
Creates a new payment account for the user associated with the access token used to make this call.
If reference_id is passed, it MUST be unique for the application/user pair or an error will be returned.
NOTE: You cannot create an account with the word 'wepay' in it.
This is to prevent phishing attacks.
Arguments:
Parameter |
Required |
Type |
Description |
name |
Yes |
String (255 chars) |
The name of the account you want to create. |
description |
Yes |
String (65535 chars) |
The description of the account you want to create. |
reference_id |
No |
String (255 chars) |
The reference id of the account. Can be any string, but must be unique for the application/user pair. |
type |
No |
String (255 chars) |
The type of account you are creating. Can be "nonprofit", "business", or "personal". |
image_uri |
No |
String (2083 chars) |
The uri for an image that you want to use for the accounts icon. This image will be used in the co-branded checkout process. |
gaq_domains |
No |
Array |
An array of Google Analytics domains associated with the account. See the analytics tutorial for more details. |
theme_object |
No |
Object |
The theme object you want to be used for account's checkout flows, withdrawal flows, and emails. See the customization tutorial for more details. |
mcc |
No |
Integer (64 bits) |
The mcc code that is relevant to the type of account this is. See the mcc reference page for more information. |
callback_uri |
No |
String (2083 chars) |
The uri that will receive IPNs for this account. You will receive an IPN whenever the account is verified or deleted. |
country |
No |
String (2 chars) |
The account's country of origin 2-letter ISO code (e.g. 'US') |
currencies |
No |
Array |
Array of supported currency strings for this account (e.g. ["USD"]) Only "USD" is supported for now. |
Example:
{
"name":"Example Account",
"description":"This is just an example WePay account.",
"reference_id":"abc123",
"image_uri":"https://stage.wepay.com/img/logo.png",
"country": "US",
"currencies": ["USD"]
}
Response:
Response |
Type |
Description |
account_id |
Integer (64 bits) |
The unique ID of the account. |
account_uri |
String (2083 chars) |
A uri that corresponds to the account's page on WePay. Do not store the returned URI on your side as it can change. |
Example:
{
"account_id":12345,
"account_uri":"https://stage.wepay.com/account/12345"
}
/account/modify
Updates the specified properties.
If reference_id is passed, it must be unique for the user/application pair.
Arguments:
Parameter |
Required |
Type |
Description |
account_id |
Yes |
Integer (64 bits) |
The unique ID of the account you want to modify. |
name |
No |
String (255 chars) |
The name for the account. |
description |
No |
String (65535 chars) |
The description for the account. |
reference_id |
No |
String (255 chars) |
The reference id for the account. Can be any string, but must be unique for the application/user pair. |
image_uri |
No |
String (2083 chars) |
The uri for an image that you want to use for the accounts icon. This image will be used in the co-branded checkout process. |
gaq_domains |
No |
Array |
The array of Google Analytics domains to be associated with the account. An empty array will remove all the Google Analytics domains previously associated with the account. See the analytics tutorial for more details. |
theme_object |
No |
Object |
The theme object you want to be used for account's checkout flows, withdrawal flows, and emails. See the customization tutorial for more details. |
callback_uri |
No |
String (2083 chars) |
The uri that will receive IPNs for this account. You will receive an IPN whenever the account is verified or deleted. |
Example:
{
"account_id":12345,
"name":"Example Account",
"description":"This is just an example WePay account. Modify the text.",
"reference_id":"abc123",
"image_uri":"https://stage.wepay.com/img/logo.png"
}
Response:
This call will return the same response as the /account call.
/account/delete
Deletes the account specified.
The use associated with the access token used must have permission to delete the account.
An account may not be deleted if it has a balance or pending payments.
Arguments:
Parameter |
Required |
Type |
Description |
account_id |
Yes |
Integer (64 bits) |
The unique ID of the account you want to delete. |
reason |
No |
String (255 chars) |
Reason for deleting the account. |
Example:
{
"account_id":12345
}
Response:
Response |
Type |
Description |
account_id |
Integer (64 bits) |
The unique ID of the account that was successfully deleted. |
state |
String (255 chars) |
The state of the account. |
Example:
{
"account_id":12345,
"state":"deleted"
}
/account/balance
Gets the balance for the account.
Arguments:
Parameter |
Required |
Type |
Description |
account_id |
Yes |
Integer (64 bits) |
The unique ID of the account you want to get the balance of. |
Example:
{
"account_id":12345
}
Response:
Response |
Type |
Description |
pending_balance |
Decimal (64 bits) |
The pending balance of the account in dollars. Note that this is the balance amount including both pending and completed transactions (in other words it is the balance after all pending transactions have cleared). |
available_balance |
Decimal (64 bits) |
The actual amount of money in dollars that has cleared and is available to the account. |
pending_amount |
Decimal (64 bits) |
The actual amount of money in dollars that is pending. |
reserved_amount |
Decimal (64 bits) |
The actual amount of money in dollars that is reserved and is not available for withdrawal (ie the minimum balance). |
disputed_amount |
Decimal (64 bits) |
The actual amount of money in dollars that is currently in dispute between the payee and the payer and is not available for withdrawal. |
currency |
String (255 chars) |
The currency of the above amounts. For now this will always be USD. |
Example:
{
"pending_balance":"500",
"available_balance:"500",
"currency":"USD"
}
/account/add_bank
This call allows you to add a bank account to a specified account. It will return a URL that a user can visit to add a bank account to their account. In addition, add_bank will allow you to change bank accounts if one was previously set.
Arguments:
Parameter |
Required |
Type |
Description |
account_id |
Yes |
Integer (64 bits) |
The unique ID of the account you want to add the bank account to. |
mode |
No |
String (255 chars) |
What mode the process will be displayed in. The options are 'iframe' or 'regular'. Choose iframe if you would like to frame the process on your site. Mode defaults to 'regular'. |
redirect_uri |
No |
String (2083 chars) |
The uri the payer will be redirected to after bank account is added. |
Example:
{
"account_id":12345,
"mode":"iframe"
}
Response:
Response |
Type |
Description |
account_id |
Integer (64 bits) |
The id of the account you added the bank account to. |
add_bank_uri |
String (2083 chars) |
The URI to add the bank account to the specified account id. Do not store the returned URI on your side as it can change. |
Example:
{
"account_id":12345,
"add_bank_uri:"http://stage.wepay.com/api/account_add_bank/12345"
}
/account/set_tax
This call lets you set tax rates for an account that will be applied to checkouts created for this account.
Taxes are only applied on a checkout if the charge_tax parameter is set to true when the checkout is created.
Arguments:
Parameter |
Required |
Type |
Description |
account_id |
Yes |
Integer (64 bits) |
The unique ID of the account you want to add tax rates to. |
taxes |
Yes |
Array |
An array of tax JSON objects. See below for the format tax objects should take. |
Example:
{
"account_id":12345,
"taxes":
[
{"percent":10,"country":"US","state":"CA","zip":"94025"},
{"percent":7, "country":"US","state":"CA"},
{"percent":5, "country":"US"}
]
}
When determining taxes to be applied, we will use the most specific match we could find.
So for the example above, if the shipping address provided by the payer matches country = US, state = CA and zip = 94025, the tax rate will be 10%.
For any addresses in California with non-94025 zip's the tax rate will be 7%.
For any addresses in the rest of the USA, not in California, the rate will be 5%.
For any addresses not in the USA, the tax rate will be 0% (as no tax was specified).
Response:
Response |
Type |
Description |
taxes |
Array |
A copy of the taxes argument that you passed in the request. |
Example:
[
{"percent":10,"country":"US","state":"CA","zip":"94025"},
{"percent":7, "country":"US","state":"CA"},
{"percent":5, "country":"US"}
]
/account/get_tax
This call lets you get the tax rates for an account.
They will be in the same format as detailed in the /account/set_tax call.
Arguments:
Parameter |
Required |
Type |
Description |
account_id |
Yes |
Integer (64 bits) |
The unique ID of the account you want to get the tax tables of. |
Example:
{
"account_id":12345
}
Response:
Response |
Type |
Description |
taxes |
Array |
The tax tables for the account. |
Example:
[
{"percent":10,"country":"US","state":"CA","zip":"94025"},
{"percent":7, "country":"US","state":"CA"},
{"percent":5, "country":"US"},
]